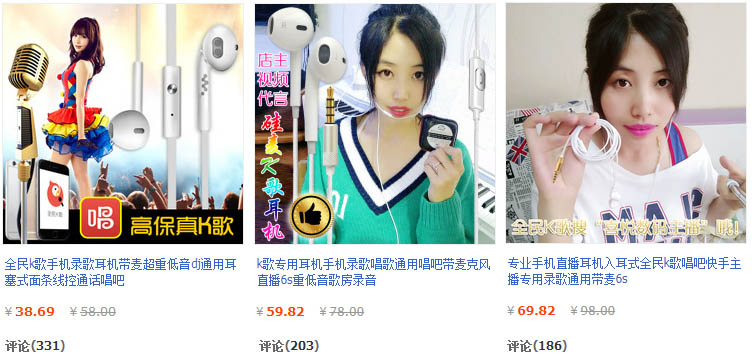
库下载地址:
https://github.com/Bodmer/TFT_eSPI
NodeMCU ESP8266-12 针脚连接方式:
// Display SDO/MISO to NodeMCU pin D6 (or leave disconnected if not reading TFT)
// Display LED to NodeMCU pin VIN (or 5V, see below)
// Display SCK to NodeMCU pin D5
// Display SDI/MOSI to NodeMCU pin D7
// Display DC (RS/AO)to NodeMCU pin D3
// Display RESET to NodeMCU pin D4 (or RST, see below)
// Display CS to NodeMCU pin D8 (只有一个屏幕也可以接GND节省一个脚)
// Display GND to NodeMCU pin GND (0V)
// Display VCC to NodeMCU 5V or 3.3V
其他板子在User_Setup.h修改即可:
//对于NodeMCU-使用pin_Dx形式的管脚号,其中Dx是NodeMCU管脚名称
//#define TFT_CS PIN_D8 // 芯片选择控制引脚D8
#define TFT_DC PIN_D3 // Data Command control pin
//#define TFT_RST PIN_D4 // 复位引脚(可以连接到NodeMCU RST,见下一行)
#define TFT_RST -1 // 如果显示器复位连接到NodeMCU RST或3.3V,则将TFT-RST设置为-1
下面是一个仿真时钟例程:
#include <SPI.h>
#include <TFT_eSPI.h> // Hardware-specific library
#define TFT_GREY 0x5AEB
TFT_eSPI tft = TFT_eSPI(); // Invoke custom library
float sx = 0, sy = 1, mx = 1, my = 0, hx = -1, hy = 0; // Saved H, M, S x & y multipliers
float sdeg=0, mdeg=0, hdeg=0;
uint16_t osx=120, osy=120, omx=120, omy=120, ohx=120, ohy=120; // Saved H, M, S x & y coords
uint16_t x0=0, x1=0, yy0=0, yy1=0;
uint32_t targetTime = 0; // for next 1 second timeout
static uint8_t conv2d(const char* p); // Forward declaration needed for IDE 1.6.x
uint8_t hh=conv2d(__TIME__), mm=conv2d(__TIME__+3), ss=conv2d(__TIME__+6); // Get H, M, S from compile time
boolean initial = 1;
void setup(void) {
tft.init();
tft.setRotation(0);
tft.fillScreen(TFT_GREY);
tft.setTextColor(TFT_WHITE, TFT_GREY); // Adding a background colour erases previous text automatically
// Draw clock face
tft.fillCircle(120, 120, 118, TFT_GREEN);
tft.fillCircle(120, 120, 110, TFT_BLACK);
// Draw 12 lines
for(int i = 0; i<360; i+= 30) {
sx = cos((i-90)*0.0174532925);
sy = sin((i-90)*0.0174532925);
x0 = sx*114+120;
yy0 = sy*114+120;
x1 = sx*100+120;
yy1 = sy*100+120;
tft.drawLine(x0, yy0, x1, yy1, TFT_GREEN);
}
// Draw 60 dots
for(int i = 0; i<360; i+= 6) {
sx = cos((i-90)*0.0174532925);
sy = sin((i-90)*0.0174532925);
x0 = sx*102+120;
yy0 = sy*102+120;
// Draw minute markers
tft.drawPixel(x0, yy0, TFT_WHITE);
// Draw main quadrant dots
if(i==0 || i==180) tft.fillCircle(x0, yy0, 2, TFT_WHITE);
if(i==90 || i==270) tft.fillCircle(x0, yy0, 2, TFT_WHITE);
}
tft.fillCircle(120, 121, 3, TFT_WHITE);
// Draw text at position 120,260 using fonts 4
// Only font numbers 2,4,6,7 are valid. Font 6 only contains characters [space] 0 1 2 3 4 5 6 7 8 9 : . - a p m
// Font 7 is a 7 segment font and only contains characters [space] 0 1 2 3 4 5 6 7 8 9 : .
tft.drawCentreString("Time flies",120,260,4);
targetTime = millis() + 1000;
}
void loop() {
if (targetTime < millis()) {
targetTime += 1000;
ss++; // Advance second
if (ss==60) {
ss=0;
mm++; // Advance minute
if(mm>59) {
mm=0;
hh++; // Advance hour
if (hh>23) {
hh=0;
}
}
}
// Pre-compute hand degrees, x & y coords for a fast screen update
sdeg = ss*6; // 0-59 -> 0-354
mdeg = mm*6+sdeg*0.01666667; // 0-59 -> 0-360 - includes seconds
hdeg = hh*30+mdeg*0.0833333; // 0-11 -> 0-360 - includes minutes and seconds
hx = cos((hdeg-90)*0.0174532925);
hy = sin((hdeg-90)*0.0174532925);
mx = cos((mdeg-90)*0.0174532925);
my = sin((mdeg-90)*0.0174532925);
sx = cos((sdeg-90)*0.0174532925);
sy = sin((sdeg-90)*0.0174532925);
if (ss==0 || initial) {
initial = 0;
// Erase hour and minute hand positions every minute
tft.drawLine(ohx, ohy, 120, 121, TFT_BLACK);
ohx = hx*62+121;
ohy = hy*62+121;
tft.drawLine(omx, omy, 120, 121, TFT_BLACK);
omx = mx*84+120;
omy = my*84+121;
}
// Redraw new hand positions, hour and minute hands not erased here to avoid flicker
tft.drawLine(osx, osy, 120, 121, TFT_BLACK);
osx = sx*90+121;
osy = sy*90+121;
tft.drawLine(osx, osy, 120, 121, TFT_RED);
tft.drawLine(ohx, ohy, 120, 121, TFT_WHITE);
tft.drawLine(omx, omy, 120, 121, TFT_WHITE);
tft.drawLine(osx, osy, 120, 121, TFT_RED);
tft.fillCircle(120, 121, 3, TFT_RED);
}
}
static uint8_t conv2d(const char* p) {
uint8_t v = 0;
if ('0' <= *p && *p <= '9')
v = *p - '0';
return 10 * v + *++p - '0';
}